Introductions:
Sometimes when computing machines are becoming smaller and smaller,we cant deny the fact that its not only the processing capabilities that we must consider , but also the capacity to store data.Since we have discussed awhile ago the speed and efficiency of this small machine/s,let's also tackle ways on how to use them as client or server to save bulk of data.Traditionally , data logging in an embedded system should have a third party connector/s or hook-up interface linked to the computers or servers before micro-controllers are able to store bulked signals or raw data .A good example was MCU gathered signals which are fed to the serial port of a PC(server) then parse via PHP and dumped those data in mysql.Another example is dumping all data at the specified ROM built in to the MCU, but the former was a better recommendation.
Just recently, I've came up to a data logger system design , comprises of different transducers which are capable of detecting digital and analog signals considered as critical parameters inside the Data Center.To name those signals: light, humidity,temperature,sounds,distance and etc. Our Arduino functions as a controller to gather data , and its embedded program measures the equivalent units to represent the scale of data sense by these transducers.
In this scratch article, we will introduce a new mysql connector ported by Dr. Chuck on his blog http://drcharlesbell.blogspot.com/2013/04/introducing-mysql-connectorarduino_6.html
.We've also used Wiznet Ethernet Shield available at the local store owned by "ThinkBox" (http://store.mytinkbox.com) ,you can order the module on-line by the way. So our contribution will be to utilize, maximize its given functions and procedure just like an ordinary SQL are coded and give an example on programming it.You may try to explore and find out how it simplifies database in an embedded systems.
Requirements:Just recently, I've came up to a data logger system design , comprises of different transducers which are capable of detecting digital and analog signals considered as critical parameters inside the Data Center.To name those signals: light, humidity,temperature,sounds,distance and etc. Our Arduino functions as a controller to gather data , and its embedded program measures the equivalent units to represent the scale of data sense by these transducers.
In this scratch article, we will introduce a new mysql connector ported by Dr. Chuck on his blog http://drcharlesbell.blogspot.com/2013/04/introducing-mysql-connectorarduino_6.html
.We've also used Wiznet Ethernet Shield available at the local store owned by "ThinkBox" (http://store.mytinkbox.com) ,you can order the module on-line by the way. So our contribution will be to utilize, maximize its given functions and procedure just like an ordinary SQL are coded and give an example on programming it.You may try to explore and find out how it simplifies database in an embedded systems.
Mysql Connector
Mysql Server on PC
Arduino2560 Mega
Wiznet Ethernet Shield (http://store.mytinkbox.com or http://www.e-gizmo.com/ )
Source: Hi-Techno Barrio http://code.google.com/p/hi-techno-barrio/downloads/list
Objectives:
(1)To enhance the capabilities of microprocessor and macro-processor as clients in a data base system.
(2) To test new Mysql connector
Methodologies:
1) Download Mysql connector
https://launchpad.net/mysql-arduino
2) Unzip the Mysql connector zip files
root@localhost# unzip mysql_connector.zip
3) Copy the files inside the Arduino library folder
root@localhost# mv mysql_connector MYSQL
root@localhost# cp -r MYSQL /usr/share/arduino/libraries
root@localhost# cp -r sha1 /usr/share/arduino/libraries
4) Cut and paste the sample code given in the Arduino IDE
root@localhost# arduino dcms.ino
5) Compile the code with your preferred Arduino2560
Note:
5.1) Select the proper baud rate (serial sets to 115200 kbs)
5.2) Just point mouse to the arrows and functions will be highlighted,click the arrows for compiling and loading
5.3) Choose the preferred sensors function in the sample program
6) Design data schema (Mysql)
root@ localhost# mysql -u root -p
6.1) create mysql database
mysql> create dcmsDB;
6.2) display mysql database
mysql> show databases;
6.3) choose mysql databse
mysql> use dcmsDB;
6.4) create table in mysql
mysql> CREATE TABLE `dcmsData` (
`sensorid` int(11) NOT NULL AUTO_INCREMENT,
`Humidity` FLOAT DEFAULT NULL,
`Light` INTEGER ,
`Noise` INTEGER DEFAULT NULL,
`Smoke` INTEGER DEFAULT NULL,
`Temperature` FLOAT DEFAULT NULL,
`AC` INTEGER DEFAULT NULL,
`DayTime` DATETIME DEFAULT NULL,
PRIMARY KEY (`sensorid`),
UNIQUE KEY `rowid_UNIQUE` (`sensorid`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
6.5) insert row data mysql table
mysql> INSERT INTO dcmsData (Humidity,Light,Noise,Smoke,Temperature,AC,DayTime) values('100','200','300','400','500','600',NOW());
6.6) display table structure in mysql
mysql> describe dcmsData
6.7) display data
mysql> SELECT * FROM dcmsData;
7) Just to give an overview , here are some parts of the program:
(6.1) Include files
#include "NewPing.h"
#include "autonomos.h"
#include "webcontrol.h"
#include "udpsend.h"
#include "DHT.h"
// include files for mysql
#include "SPI.h"
#include "Ethernet.h"
#include "sha1.h"
#include "mysql.h"
6.2) /* Setup for Ethernet Library */
/* Setup for Ethernet Library */
byte mac_addr[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
IPAddress server_addr(192,168,1,2);
IPAddress ip(192,168,1,177);
IPAddress gateway(192,168,1, 1);
IPAddress subnet(255, 255, 255, 0);
char sqlbuf[128];
char sqlDbase[] = " USE dcmsDB";
6.3) /*Setup for MySQL */
unsigned int mysqlPort=3306;
Connector my_conn; // The Connector/Arduino reference
char user[] = "root";
char password[] = "Igorotzki";
boolean sqlconnect= false;
(6.4) Mysql procedures
void mysqlBegin()
{
delay(350);
Serial.println("connecting MySQL Server..");
if (my_conn.mysql_connect(server_addr, mysqlPort, user, password))
{
sqlconnect=true;
Serial.println("Query Success!");
delay(150);
my_conn.cmd_query(sqlDbase);
}
else
{
Serial.println("Connection failed.");
}
}
// sending data to mysql
void mysqldata()
{
// uncomment to use the given functions
// tmp = temperature();
// ht = humidity();
// smk = smoke();
// ns = noise();
// lt = light();
// ac = ACData();
if (sqlconnect==true)
{
delay(150);
//"INSERT INTO dcmsData (Humidity,Light,Noise,Smoke,Temperature,AC,DayTime) values('100','200','300','400','500','600',NOW()) ";
sprintf(sqlbuf, "INSERT INTO dcmsData (Humidity,Light,Noise,Smoke,Temperature,AC,DayTime) values ('%f','%d','%d','%d','%d','%f',NOW())",ht,lt,ns,smk,tmp,ac) ;
my_conn.cmd_query(sqlbuf);
Serial.println("Data stored!");
}
} // end mysql...!
6.5)
// main program
Void loop()
{
mysqldata();
range(1);
robotCommand("independent");
cameraPosition();
}
6.6)
If you are interested with the complete code of this techno-blog, please include your e-mail account in the comment portion!
Details(1) Data logging (DHT11,LM35,LDR,Sound,URF)
Details(2) Mysql Connector download site
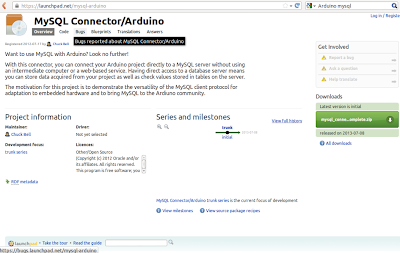
Details(3) Mysql connector and its compilation
Details(4) Unzip the mysql connector files
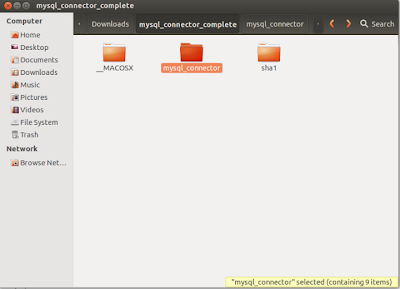
Details(5) Successful compilation of Mysql connector using Ubuntu
Details(6) Mysql shema ->database ->tables
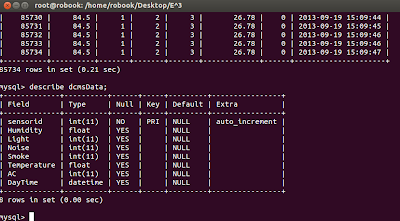
Details(7) Php code
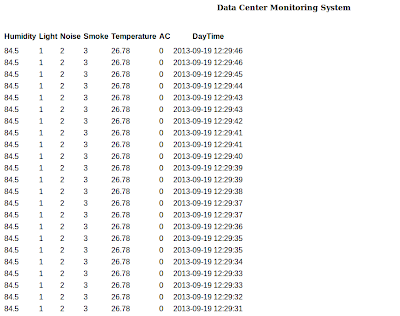
Details(8): Web page Display (PHP)
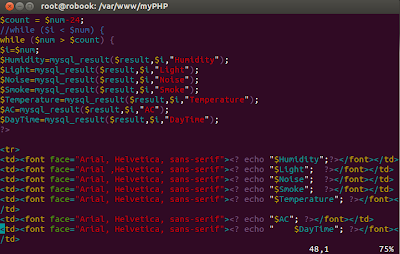
Summary:
Trouble(1)Serial.print function ("Error 255=." and then "Connection failed")
Shooting:
GRANT ALL ON *.* TO 'root'@'%' IDENTIFIED BY 'your_password';
Trouble(2) Using database
Shooting: Please add the following procedure
my_conn.cmd_query("USE dcmsDB);
Trouble(3)
in sha1.h virtual void write(uint8_t);
error: conflicting return type specified for 'virtual void Sha1Class::write(uint8_t)'
do you know how to fix it?
Shooting:
Apply the diff included with the source code.
Trouble(4) Declaring floating point in mysql query
Shooting:
float t = do_something();
char buf[128];
sprintf(buf, "INSERT INTO test.motion VALUES (NULL, '%f')", t);
my_conn.cmd_query(buf);
Conclusions:
So we have embedded mysql in MCU
TO E^3
ReplyDeleteCompile successfully but the database of the values 0
It has something to do with mysql data type (Float/Decimal point) ,or better convert float type into integer in Arduino C.
DeleteHere is the thread for that- http://forum.arduino.cc/index.php/topic,44340.0.html
Hope this helps
Cheers!
E^3
DeleteHi folks,
I've done it using "dtostrf()" function below is the sample code
Cheers!
E^3
// sending data to mysql
void mysqldata()
{
// uncomment to use the given functions
// tmp = temperature();
// ht = humidity();
// smk = smoke();
// ns = noise();
// lt = light();
// ac = ACData();
if (sqlconnect==true)
{
delay(150);
dtostrf(ht,1,2,htStr);
dtostrf(tmp,1,2,tmpStr);
//"INSERT INTO dcmsData (Humidity,Light,Noise,Smoke,Temperature,AC,DayTime) values('100','200','300','400','500','600',NOW()) ";
sprintf(sqlbuf, "INSERT INTO dcmsData (Humidity,Light,Noise,Smoke,Temperature,AC,DayTime) values ('%s','%d','%d','%d','%s','%d',NOW())",htStr,lt,ns,smk,tmpStr,ac) ;
//char SQL[] = sqlbuf +"NOW(()";
my_conn.cmd_query(sqlbuf);
Serial.println("Data stored!");
}
} // end mysql...!
How to Monitor window displays this information is sent to the database what??
DeleteWritten to the database because the current temperature, to a database query are displayed 0
So how to do this - >> Serial.println ();
To show the value before sending it to the database???
sprintf (buf, "INSERT INTO tempdb.table3 (temp_temp1) values ('% f')", t);
Hi folk (What's your name :) )
DeleteIf you have read the blog,you will know that it has a multiple sensors,and you can see the outputs using serial monitor(Arduino IDE) and via web using PHP.
Here is the link where you can download all the files
http://code.google.com/p/hi-techno-barrio/downloads/detail?name=E^3.zip&can=2&q=
Hope this helps you.
E^3
Philippines
The above problem has not been solved yet
DeleteBelow is a small mention (I'm not using arduino
# include
# include )
I would like to say that I use another way to send temperature data to the database are sent to the database, but temperatures are shown integers
Such as: 26oC so I want to ask, how to let him send out the float, such as: 26.55oC
The following is to create a database format settings:
# --------- Name ------------ Type ----- empty ---- Default
3 ---- temp_temp1 --------- float -------- yes ------ NULL
sensors.requestTemperatures ();
float T = sensors.getTempCByIndex (0);
server.write (T);
Serial.println (T);
Control room window to see a floating point value, but to look at the database is an integer
This:
server.write (T);
I had to check his grammar only
server.write (data)
data: the value to write (byte or char)
So I would like to ask how he can display floating point
Hi 謝佳偉,
DeleteHere is what I did
1) You need to convert float into string
ex:
/* Arduino IDE /C program side */
char strFloat[10];
Float T= sensonrs.getTempCByIndex(0);
/* Take Note!
You've have converted float into string type just to display it */
dtostrf(T,1,2,strFLoat);
Serial.println('%s',strFloat)
Server.write('%s',strFloat)
2) In your mysql schema,just declare the type as float; please take a look at the details # 6 ,7 & 8 for references.
Cheers!
E^3
24 void loop(){
Delete26 EthernetClient client =server.available();
27 if(client >= 0)
28{
29 sensors.requestTemperatures();
30 float T= sensors.getTempCByIndex(0);
31 dtostrf(T,1,2,strFLoat);
32 Serial.print("Temperature \t");
33 Serial.println('%s',strFloat);
34 server.write('%s',strFloat);
35 delay(1000);
36}
------------------------------------------------------
appear Error
Temperature_Server0919.ino: In function 'void loop()':
Temperature_Server0919:31: error: 'strFLoat' was not declared in this scope
Temperature_Server0919:33: error: invalid conversion from 'char*' to 'int'
Temperature_Server0919:33: error: initializing argument 2 of 'size_t Print::println(int, int)'
Temperature_Server0919:34: error: invalid conversion from 'int' to 'const uint8_t*'
Temperature_Server0919:34: error: initializing argument 1 of 'virtual size_t EthernetServer::write(const uint8_t*, size_t)'
Temperature_Server0919:34: error: invalid conversion from 'char*' to 'size_t'
Temperature_Server0919:34: error: initializing argument 2 of 'virtual size_t EthernetServer::write(const uint8_t*, size_t)'
Hi brother,
Delete0) Check your typo error/proper letter /spelling
1) please add this in your header ..
#include "stdlib.h"
2) declare the strFloat
char strFloat[10];
3) you may try
Serial.println('%s',strFloat);
/* maybe your server.write(strFloat)
server.write(strFloat);
cheers!
E^3
30{
Delete31 sensors.requestTemperatures();
32 float T= sensors.getTempCByIndex(0);
33 Serial.print("Temperature \t");
34 Serial.println('%s',strFloat);
35 server.write(strFloat);
36 delay(1000);
37}
----------------------------------------------------------------------------------------------------------
appear Error
Temperature_Server0919.ino: In function 'void loop()':
Temperature_Server0919:34: error: invalid conversion from 'char*' to 'int'
Temperature_Server0919:34: error: initializing argument 2 of 'size_t Print::println(int, int)'
Do not understand what you mean??
Delete
DeleteHi 謝佳偉
//replace...
Serial.println('%s',strFloat);
// with...
Serial.printlin(strFloat);
You can understand me now-your problem is solved perhaps
E^3
void loop(){
DeleteEthernetClient client =server.available();
if(client >= 0)
{
sensors.requestTemperatures();
float T= sensors.getTempCByIndex(0);
Serial.print("Temperature \t");
Serial.println(strFloat);
server.write(strFloat);
delay(1000);
}
-----------------------------------------------------------------------------------------
Windows has been compiled successfully monitor displays [Temperature]
Database did not show any increase in, the temperature values
Hi 謝佳偉,
Delete1) Please declare "strFloat"
char strFloat[10];
2) Add float to string conversion
dtostrf(T,1,2,strFloat);
//-----------------------------------------------------
char strFloat[10];
void loop(){
EthernetClient client =server.available();
if(client >= 0)
{
sensors.requestTemperatures();
float T= sensors.getTempCByIndex(0);
dtostrf(T,1,2,strFloat);
Serial.print("Temperature \t");
Serial.println(strFloat);
server.write(strFloat);
delay(1000);
}
//---------------------------------------------------------
Cheers!
E^3
[Two different things]
DeleteReply to this above you have a decimal point
[Another thing]
My arduino temperature sensing + database
Direct manual transmission value is written to the database in the database can be dangerous
But let the temperature value is automatically written still not resolved
I really need your mailbox, I want to send my temperature code into your mailbox, I hope you can help me with me for a long period of time, the temperature values can not be thrown into the database, please!!
DeleteMy mailbox is aaa47022003@gmail.com
If do not want to open the case, please send a letter to indicate your name, I can contact you on
hi 謝佳偉,
DeleteCan you please give me the following detail?
1) Your hardware setup
2) Your project design
3) Your Gantt Chart
4) Your application to this
Thanks
E^3
Can you please give me the following detail?
Delete1) Your hardware setup: arduino-1.5.2-windows
2) Your project design:
php + arduino temperature sensing works so arduino which can be used in your code to the database,
And the temperature values thrown into a database, php web crawler is doing database temperature values are displayed on the form
Recently there have been doing PHP + arduino website, because I found one, but the site has gone off the arduino temperature values sent to the database, so I want to pass values to the database arduino, so to turn off the page can still operate as usual
3) Your Gantt Chart: the card can not be [Auto] pass values to the database, [it is can be transferred manually to the database]
4) Your application to this
Database: tempdb
DeleteTable: table3
Field Name Order: temp_id -----> temp_date -----> temp_temp1
-------------------------------------------------- -------------------------------------------------- ---------
I have always felt a problem here, but will not go to solve
sprintf (buf, "INSERT INTO tempdb.table3 (temp_temp1) values ('% f', T)");
my_conn.cmd_query (buf);
Deletehi
Have you not tried this..
http://code.google.com/p/hi-techno-barrio/downloads/detail?name=E^3.zip&can=2&q=
I included all the source code there : mysql,php,arduino (code & libraries).You can use it.
Thanks
E^3
This I have to see, but still will not use
DeleteMicrocontroller Embedded Design: Arduino Mysql Connector (Arduino On-Line/Real-Time Database) >>>>> Download Now
Delete>>>>> Download Full
Microcontroller Embedded Design: Arduino Mysql Connector (Arduino On-Line/Real-Time Database) >>>>> Download LINK
>>>>> Download Now
Microcontroller Embedded Design: Arduino Mysql Connector (Arduino On-Line/Real-Time Database) >>>>> Download Full
>>>>> Download LINK nn
What is the dcms.ino??? Where can I get it?
ReplyDeletebpg_92@hotmail.com
Delete
DeleteHi Amigo,
Here is the link where you can download all the files
http://code.google.com/p/hi-techno-barrio/downloads/detail?name=E^3.zip&can=2&q=
Hope this helps you.
E^3
And I can't find these libraries:
ReplyDelete#include "NewPing.h"
#include "autonomos.h"
#include "webcontrol.h"
#include "udpsend.h"
#include "DHT.h"
Hi Benjamine,
ReplyDeleteJust repackaging it , for awhile :)
Ok, thanks!
DeleteHere is the link where you can download all the files
Deletehttp://code.google.com/p/hi-techno-barrio/downloads/detail?name=E^3.zip&can=2&q=
Hi E^3,
ReplyDeleteI need your help!
I can store directly in my sql server the values that i set, but i want to store values from photocell, can you help me?
That's my code:
int photocellPin = 0;
int photocellReading;
void loop(void) {
photocellReading = analogRead(photocellPin);
void mysqldata()
{
if (sqlconnect==true)
{
delay(150);
sprintf(sqlbuf, "INSERT INTO dcmsdata (Humidity) values ('1');") ;
my_conn.cmd_query(sqlbuf);
Serial.println("Data stored!");
}
}
// Instead of Value '1' i want to put photocell value, how i set this variable?
Best Regards
Hi Darkmilhas,
ReplyDeletevoid mysqldata()
{
if (sqlconnect==true)
{
delay(150);
sprintf(sqlbuf, "INSERT INTO dcmsdata (Humidity) values ('%d')",photocellReading) ;
my_conn.cmd_query(sqlbuf);
Serial.println("Data stored!");
}
}
Cheers!
E^3
Hi E^3,
DeleteIt work's!! :D Now i have another situation, i think is related to the conversions, float to string, i read the posts before but i still a little confuse.
Mysql temperature column is (FLOAT). I cannot save in db the temperature value, temperature value i can see that have this format: 23.10
Error: 57 = Data truncated for column 'Temperature' at row 1.
So, i will try give my code:
char tmpStr[10];
void mysqldata()
{
if (sqlconnect==true)
{
delay(150);
dtostrf(tmp,1,2,strFloat);
sprintf(sqlbuf, "INSERT INTO dcmsdata (Light,Temperature) values ('%d','%s')",photocellReading,tmp) ;
my_conn.cmd_query(sqlbuf);
Serial.println("Data stored!");
}
} // end mysql...!
void loop()
{
float getTemperature(){
//all remaining code for get temperature
}
// In serial monitor temperature is displayed but in database doesn't work.
Tnk you for your help! Best regards
Hi E^3,
DeleteCan you help me with this?
Tnk you very much!
Hi Darkmilhas,
DeleteCan you post here your mysql schema?
Lets see what we can do.. :)
E^3
Heloo E^3:
ReplyDelete`sensorid` int(11) NOT NULL AUTO_INCREMENT,
`Humidity` float DEFAULT NULL,
`Light` int(11) DEFAULT NULL,
`Noise` int(11) DEFAULT NULL,
`Smoke` int(11) DEFAULT NULL,
`AC` int(11) DEFAULT NULL,
`DayTime` datetime DEFAULT NULL,
`Temperature` float DEFAULT NULL,
PRIMARY KEY (`sensorid`),
UNIQUE KEY `rowid_UNIQUE` (`sensorid`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Regards from Portugal! ;)
Hi Darkmilhas,
DeletePlease check the accessibility of mysql this time
Shooting:
GRANT ALL ON *.* TO 'root'@'%' IDENTIFIED BY 'your_password';
Hope this helps...
Cheers!
E^3
Hi there, I seriously desire to declare that I came across your post and found it to be interesting.Thanks for sharing such a valuable information and this information really helpful for us.
ReplyDeleteembedded hardware board design || hardware prototypes design
Hi einfochips,
ReplyDeleteYou can find more interesting post /article in my blog, please feel free to comment question and subscribe to it.
Thanks & more power too.
E^3
Philippines
i am interested in the complete code, email is codepoet272@gmail.com
ReplyDeleteHi broter,
ReplyDeletehere is the code E^3.Zip on " Data Center Monitoring System"
http://code.google.com/p/hi-techno-barrio/downloads/list
Thanks
E^3
hi! i have no problem compiling it. I uploaded the sketch successfully but then the serial monitor prints connection failed. I am using wampserver and i placed the index.php and dbinfo.inc.php inside the www/wamp folder. i edited the password and username as "mark" and "mark" but it just won't connect to the database
ReplyDeleteHi bro,
Deleteplease check(compare/modify) the initialized IP addresses of both (host)Mysql server and arduino(client). Actually I configured it as static IP.
Hope this helps..
-E^3
Hi sir, I have a float type variable name conductance and I insert into my database like this: "INSERT INTO test (conductance) VALUES (conductance)" but the database has an empty value.
ReplyDeleteSo I tried using sprintf to convert float into string but always compile error.
Then I found dtostr method but whenever I used it the program stops at "Connecting....".
I seek your professional advice please. Thabk you.
Hi Stephanie,
DeleteA)--------Documents & Source Code-----------
here is the code E^3.Zip on " Data Center Monitoring System"
http://code.google.com/p/hi-techno-barrio/downloads/list
B)----------------How--To-------------------------------
1) Please declare "strFloat"
char strFloat[10];
2) Add float to string conversion
dtostrf(T,1,2,strFloat);
//-----------------------------------------------------
char strFloat[10];
void loop(){
EthernetClient client =server.available();
if(client >= 0)
{
sensors.requestTemperatures();
float T= sensors.getTempCByIndex(0);
dtostrf(T,1,2,strFloat);
Serial.print("Temperature \t");
Serial.println(strFloat);
server.write(strFloat);
delay(1000);
}
Thanks
E^3
Philippines
Hello E^3,
ReplyDeleteI can't put temperature value in database, i follow all comments about string and float and all trys that i make, doesn't work.
char strFloat[10];
void mysqldata()
{
float t= getTemperature();
dtostrf(t,1,2,strFloat);
Serial.println(strFloat);
if (sqlconnect==true)
{
delay(150);
sprintf(sqlbuf, "INSERT INTO dcmsdata (Light,Temperature) values ('%d','%d')",photocellReading,t) ;
my_conn.cmd_query(sqlbuf);
Serial.println("Data stored!");
}
}
//Serial monitor shows:
Light = 128
Temperature = 20.71 | Humidity = 73.40
20.70
Data stored!
__-
Database show:
Light: 128
Temperature:18350
sometimes temperature gives also negative values..
The my schema is the same that you use..
Can you help me?
tnk you
Hi Sir Darkmilhas,
DeleteHere is the code E^3.Zip on " Data Center Monitoring System"
http://code.google.com/p/hi-techno-barrio/downloads/list
Thanks
E^3
Can I install picuntu following this guide on my mk808b? I have exacly the same device like in pictures in this post: http://www.freaktab.com/showthread.php?11002-help-mk808b-clone-not-working-*noob*&p=151907&viewfull=1#post151907
ReplyDeleteHi SIr
ReplyDeletepls try this blog
http://cobecoballes-embedded.blogspot.com/2013/08/writing-image-from-mk808rk3066-in-linux.html
thanks
-E^3
Hello
ReplyDeleteMy Name is Markus from Switzerland.
Sorry for my english its very bad.
I make my own Weatherstation with tow Arduino and 433MHz funk conektion
and printing on the Web.
Its very easy to work without float.
If the Temperatur 22.5C, then multiplikation with 100.
Now, i send 2250 and write 2250 in the databank.
And divide for printing on the Web!
This is my simplay way, bat its Work.
One little trick, for lesser trouble:
After the writing in database, close the connecton with:
my_conn.disconnect();
And for a new conection, test for a old connection, and disconnect with:
if (my_conn.is_connected() != 0) my_conn.disconnect();
Hi Sir Markus,
ReplyDeleteThanks for sharing your ideas,hope to receive more trouble shooting from your projects
Cheers!
-E^3
Hy sir
ReplyDeleteI have some trouble with arduino sketch, the error is "Arduino\libraries\MYSQL\sha1.cpp:11:25: error: variable 'sha1InitState' must be const in order to be put into read-only section by means of '__attribute__((progmem))'
uint8_t sha1InitState[] PROGMEM = {"
what should i do sir?
DeleteHi Sir Yonas.
Please visit this code,
http://code.google.com/p/hi-techno-barrio/downloads/list
..and lets see if it can help when you compile the code
Thanks
-E^3
Hi, your article is very hepful to me and my ideas. but i have a question.
ReplyDeletematerials:
Arduino Mega 2650, ethernet shield, DHT22 v2 sensor to wamp and mysql db.
in many foros the problem is the connection with myql. i looking for the solution i found sha1 and mysql libreries and don't work to me. but set values in the url and press enter, the data is in the bd...
any suggest for me??
pd: Sorry for the English is not my mother language.
Greetings from Chile
Hello Sir Pablo,
DeletePlease visit this code,
http://code.google.com/p/hi-techno-barrio/downloads/list
..and lets see if it can help when you compile the code
Thanks
-E^3
Hello.
ReplyDeleteI'm with a error when i run my code.
It appears Error 5: .
Someone can help me?
Greetings from Brasil.
DeleteHi Sir Jonathan,
Please rerun your code, check some typos or follow the procedures.
If still problem occurs then say something happened.
Cheers!
-Christopher
Hi Ricardo ,
ReplyDeleteYou are welcome,
Please follow and share this blog .
Cheers!
-Christopher
this is for linux i want to connect my arduino to mysql using windows 10 so what would be the procedure plzz reply me....
ReplyDeleteHi Mitesh Naik,
DeleteThere is no problem as long as you can run mysql in your Windows 10 -its easy . 1) download the latest mysql 2) create database and 3) dump the sql file included in my tutorial
-Cheers
-E^3
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteHello, could you send me full arduino sketch please?
ReplyDeleteaggressiv@gmail.com
Hi Sir Baris Guler,
DeleteJust wait , I am looking for it.
Thanks
E^3
Philippines
Hi Baris
ReplyDeletePlease click this link
https://code.google.com/p/hi-techno-barrio/downloads/list
..and download E^3.zip
cheers!
-E^3
i've just seen the file, this was my mistake, sorry..
DeleteHello, One last question,
Deletedid you achieve to measure AC220v data? if yes, how did you du that? i couldnt find useful document or it looks there is no simple sensor to do that. Can you show me the way? or can you give me an idea?
Hi Baris,
DeleteYou can use this device-hall sensor effect
http://www.amazon.com/0-01-120A-0-5M-Split-core-Current-Transformer/dp/B005FIFT4E
Cheers!
-E^3
Philippines
This comment has been removed by the author.
DeleteHi E^3
DeleteHow can I send data from MQ 2,3,4&5 to database to MySQL and show result in LabVIEW using windows 7 64 bits
DeleteI think you can send it via port (Serial/I2C) depends on which driver do you have to display it via LabView .
Thanks
-E^3
christ3m@yahoo.fr
ReplyDeleteAll of the issues are about updating data to database from adruino... but if I wanna retrieve a specific data from the database and store it in a variable for further use, how can that be accmplished? Please enlighten me
ReplyDeleteHi Sir,
DeleteYou can use the program and try to look for the sample of mysql querying, in that way you can substitute in the code to retreive whatever data you have in the mysql database.
Hope I have answered your questions well.
Thanks
E^3
hi..
ReplyDeletecan u pls email me the code and the libraries
my email is edrisaa.turay@gmail.com
Thank you... :)
Please click this link
ReplyDeletehttps://code.google.com/p/hi-techno-barrio/downloads/list
..and download E^3.zip
cheers!
-E^3
me and my friend would redecorate ur room, and redistribute ur machines
ReplyDeleteThank you so much great work, I have Arduino Yun, I would like to store my temperature data to mysql but I don't know how?,,
ReplyDeleteCurrently I can see the temperature data on browser and it creates a graph for last 5 temperature I would like to save them in mysql from where to start I don't know:
my code for sketch:
#include
#include
#include
// Listen on default port 5555, the webserver on the Yún
// will forward there all the HTTP requests for us.
BridgeServer server;
String startString;
long hits = 0;
float lastTemp[5];
void setup() {
SerialUSB.begin(9600);
// Bridge startup
pinMode(13, OUTPUT);
digitalWrite(13, LOW);
Bridge.begin();
digitalWrite(13, HIGH);
// using A0 and A2 as vcc and gnd for the TMP36 sensor:
pinMode(A0, OUTPUT);
pinMode(A2, OUTPUT);
digitalWrite(A0, HIGH);
digitalWrite(A2, LOW);
// Listen for incoming connection only from localhost
// (no one from the external network could connect)
server.listenOnLocalhost();
server.begin();
// get the time that this sketch started:
Process startTime;
startTime.runShellCommand("date");
while (startTime.available()) {
char c = startTime.read();
startString += c;
}
}
void loop() {
// Get clients coming from server
BridgeClient client = server.accept();
// There is a new client?
if (client) {
// read the command
String command = client.readString();
command.trim(); //kill whitespace
SerialUSB.println(command);
// is "temperature" command?
if (command == "temperature") {
// get the time from the server:
Process time;
time.runShellCommand("date");
String timeString = "";
while (time.available()) {
char c = time.read();
timeString += c;
}
SerialUSB.println(timeString);
int sensorValue = analogRead(A1);
// convert the reading to millivolts:
float voltage = sensorValue * (5000.0f / 1024.0f);
// convert the millivolts to temperature celsius:
float temperature = (voltage - 500.0f) / 10.0f;
// print the temperature:
/* client.print("Current time on the Yún: ");
client.println(timeString);
client.print("
Current temperature: ");
client.print(temperature);
client.print(" °C");
client.print("
This sketch has been running since ");
client.print(startString);
client.print("
Hits so far: ");
client.print(hits);
}
*/
lastTemp[hits % 5] = temperature; for(int i=0;i<5; i++)
{ client.print(lastTemp[i]); client.print(";"); } client.println(timeString+";"+startString+";"+hits+";");
}
//client.println(timeString+";"+startString+";"+hits+";");
// Close connection and free resources.
client.stop();
hits++;
}
delay(50); // Poll every 50ms
}
Hi,
DeleteThanks for sharing.
E^3
Microcontroller Embedded Design: Arduino Mysql Connector (Arduino On-Line/Real-Time Database) >>>>> Download Now
ReplyDelete>>>>> Download Full
Microcontroller Embedded Design: Arduino Mysql Connector (Arduino On-Line/Real-Time Database) >>>>> Download LINK
>>>>> Download Now
Microcontroller Embedded Design: Arduino Mysql Connector (Arduino On-Line/Real-Time Database) >>>>> Download Full
>>>>> Download LINK